Mastering Min and Max Value Retrieval in JavaScript Arrays
Written on
Chapter 1: Introduction to Min/Max Values
In many programming scenarios, we may need to determine the minimum or maximum value of a specific property within objects contained in a JavaScript array. This article will guide you through the process of extracting these values.
To achieve this, we will utilize the map method, which allows us to create a new array that consists of the values of the desired property. By employing the Math.min and Math.max functions, we can easily find the minimum and maximum values by spreading the resulting array of values into these methods.
Section 1.1: Utilizing the Map Method
To illustrate how to obtain the min or max values, consider the following example:
const myArray = [{
id: 1,
cost: 200
}, {
id: 2,
cost: 1000
}, {
id: 3,
cost: 50
}, {
id: 4,
cost: 500
}];
const min = Math.min(...myArray.map(item => item.cost));
const max = Math.max(...myArray.map(item => item.cost));
console.log(min); // Output: 50
console.log(max); // Output: 1000
In this code snippet, we utilize myArray.map to generate an array containing the cost values from each object. Subsequently, we spread these values as arguments into Math.min and Math.max to retrieve the respective minimum and maximum values for the cost property.
Subsection 1.1.1: Visual Representation
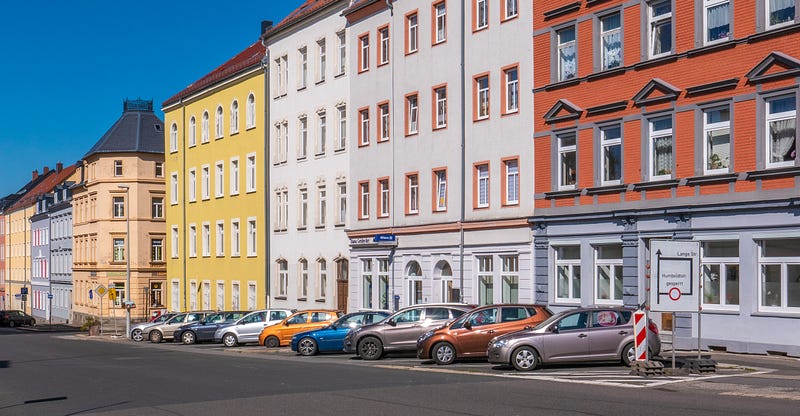
Section 1.2: Conclusion
In summary, you can efficiently determine the min or max value of a property in an array of JavaScript objects by leveraging the map method in conjunction with Math.min or Math.max. This method not only simplifies the process but also enhances readability in your code.
Chapter 2: Further Learning
For more insights on handling arrays in JavaScript, check out the following videos that delve into finding minimum and maximum values:
The first video titled "JavaScript Tip: Finding the Minimum or Maximum Value in an Array" provides a concise overview of techniques to achieve this.
The second video "How to find min and max value in an array | One Minute Javascript" offers a quick, practical demonstration of the method.
Stay updated with more content at PlainEnglish.io, and consider signing up for our free weekly newsletter. Follow us on Twitter, LinkedIn, YouTube, and Discord for ongoing discussions and resources.