Mastering JavaScript One-Liners: Your Path to Coding Greatness
Written on
Chapter 1: Introduction to JavaScript One-Liners
JavaScript one-liners are succinct expressions that execute specific tasks in a single line of code. While they may appear simple, these snippets can significantly enhance your programming prowess and impress your peers with your advanced coding abilities.
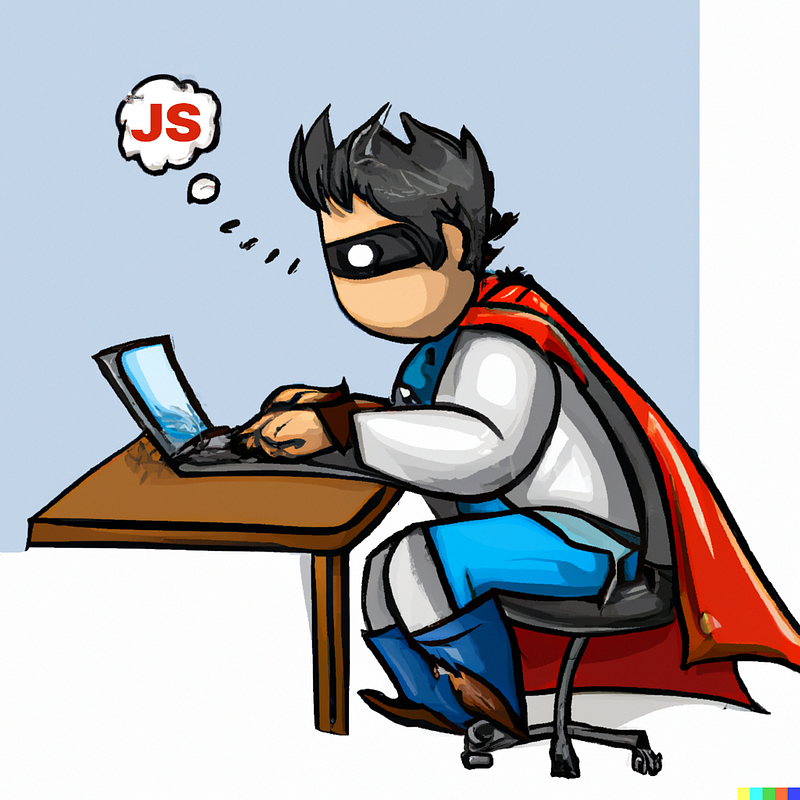
Chapter 2: Practical Applications of One-Liners
Section 2.1: Extract Unique Elements from an Array
To obtain an array devoid of duplicates, you can utilize a straightforward method. By forming a Set from the original array and converting it back to an array using the spread operator, you can easily filter out repeated values.
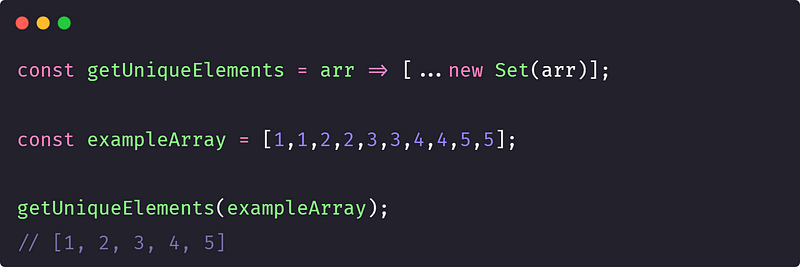
Section 2.2: Generate Random Booleans
This function returns either true or false, each with a 50% likelihood. It leverages Math.random to produce a random number between 0 and 1, subsequently evaluating if the number is greater or less than 0.5.
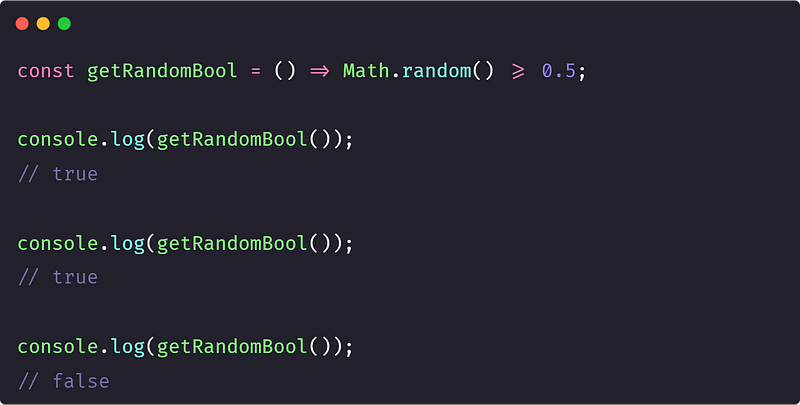
Section 2.3: Random Number Generation
You can create a function to generate a random number within a specified range, defined by minimum and maximum values.
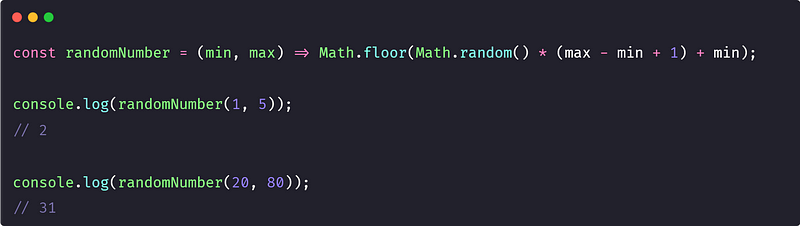
Section 2.4: Swapping Variables
Did you know it's possible to swap two variables without using a temporary variable? This can be accomplished neatly in a single line of code.
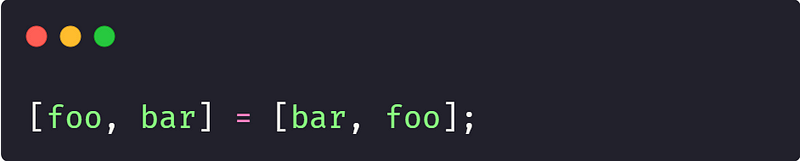
Section 2.5: Scroll to Top Functionality
Using the scrollTo(x, y) function, you can effortlessly navigate to specified coordinates, with the top coordinates set at (0,0).
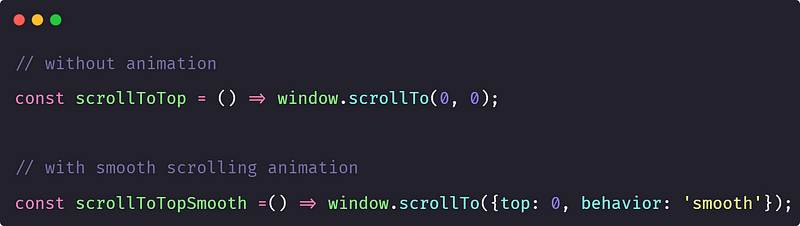
Section 2.6: Calculating Averages
You can create a getAverage function utilizing the Array.prototype.reduce() method to compute the average of numerical values in an array.
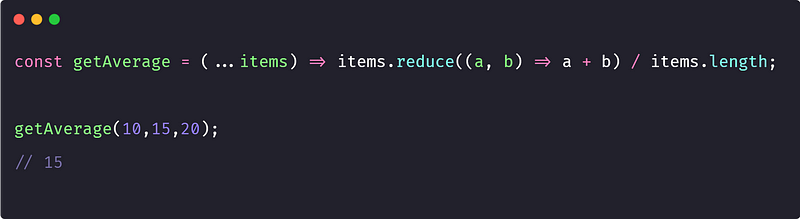
Section 2.7: Counting Characters in a String
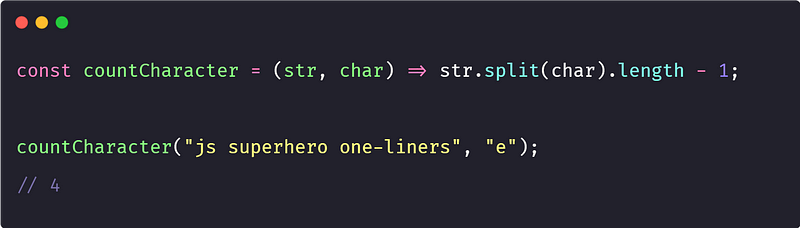
Section 2.8: Retrieving Selected Text on a Webpage
You can leverage a built-in browser method to capture the text that users select on a webpage.

Section 2.9: Cloning Arrays
There are multiple methods to clone an array; here are my two preferred techniques.
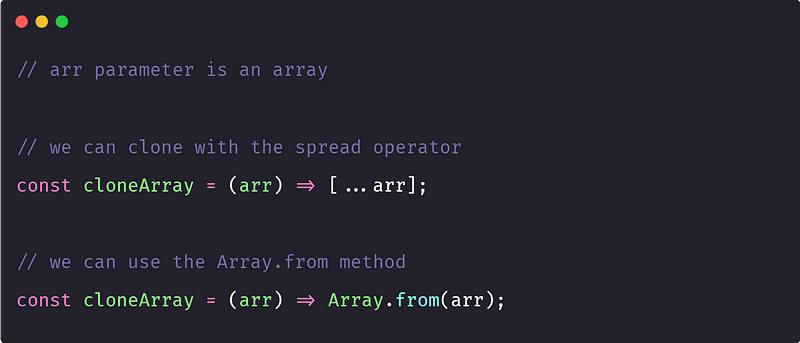
Section 2.10: Counting Value Occurrences in an Array
You can count how many times a particular value appears in an array using the array.filter method.
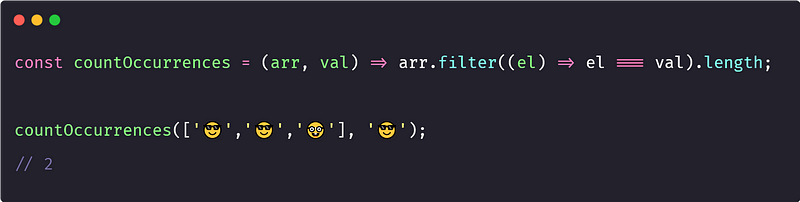
Section 2.11: Palindrome Check
A palindrome is a string that reads the same forwards and backwards.
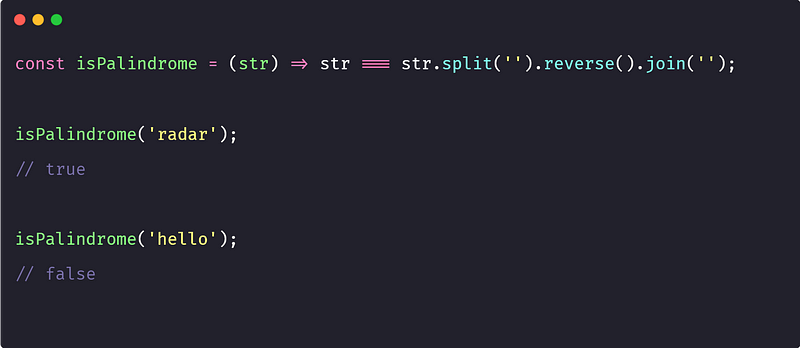
Section 2.12: Generating Random Hex Colors
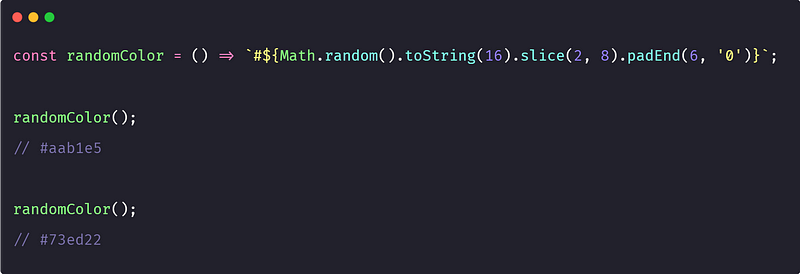
Section 2.13: Capitalizing Strings
If you prefer not to use CSS for capitalizing strings, you can achieve this with a simple one-liner.
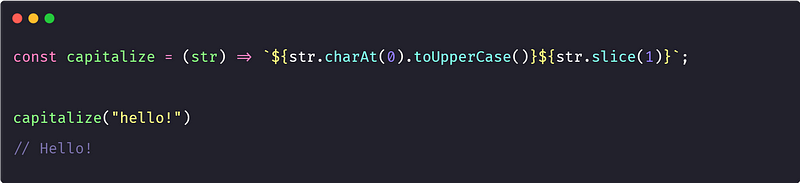
Chapter 3: Conclusion
While one-liners won't turn you into a superhero, they will definitely elevate your JavaScript skills to new heights. So dive in and start crafting code that can truly make a difference!
Explore the video titled "10 Life-Saving JavaScript One-Liners CODE LIKE A PRO 2022" to see practical implementations of these concepts in action.
In the video "15 Superhero projects in HTML5/CSS3/JavaScript! Weekly challenge review," witness how these one-liners can be applied in exciting projects.