How to Resolve the 'Invalid React Child' Error Effectively
Written on
Understanding the 'Invalid React Child' Error
When developing React applications, you might face the error stating, "Objects are not valid as a React child. If you intended to render a collection of children, use an array instead." This issue can disrupt the rendering process, but there are straightforward methods to address it.
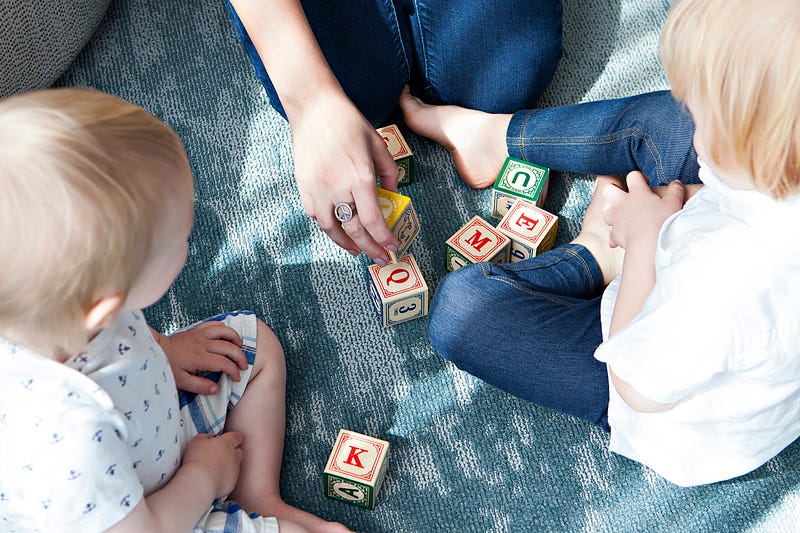
Solutions to the Error
To resolve the "Invalid React Child" error, you can utilize the map method to render an array or convert object properties to strings for rendering.
For example, consider the following code:
const arr = [1, 2, 3];
export default function App() {
return (
<div className="App">
{arr.map((num) => (
<div key={num}>{num}</div>))}
</div>
);
}
In this example, we are rendering the arr array into multiple div elements. Each element in the array is assigned a unique key for optimal performance. The values in the array (in this case, numbers) are displayed directly within the div.
If you attempt to render an object directly, you'll encounter issues. Instead, you can convert the object to a string like this:
const obj = { foo: 1, bar: 2 };
export default function App() {
return <div className="App">{JSON.stringify(obj)}</div>;
}
In this case, we use JSON.stringify to convert the object into a string for rendering. Alternatively, you can display individual properties from the object:
const obj = { foo: 1, bar: 2 };
export default function App() {
return <div className="App">{obj.foo}</div>;
}
Conclusion
In summary, the "Objects are not valid as a React child" error can be effectively addressed by rendering arrays using the map method, converting objects to strings, or displaying object properties individually.
In Plain English
We appreciate your engagement with our content! Before you leave, don't forget to show support by clapping and following the author. For more insights, visit PlainEnglish.io and subscribe to our weekly newsletter. Stay connected with us on Twitter(X), LinkedIn, YouTube, and Discord. Explore our other platforms: Stackademic, CoFeed, and Venture.