Exploring Concurrency Design Patterns in Go Programming
Written on
Introduction to Concurrency Patterns
In this article, we will delve into twelve concurrency design patterns utilized in Go, which include Select, For-Select-Done, Future, Barrier, Pipeline, Worker Pool, Fan-Out Fan-in, Producer-Consumer, Semaphore, Rate Limit, Circuit Breaker, and Publish-Subscribe.
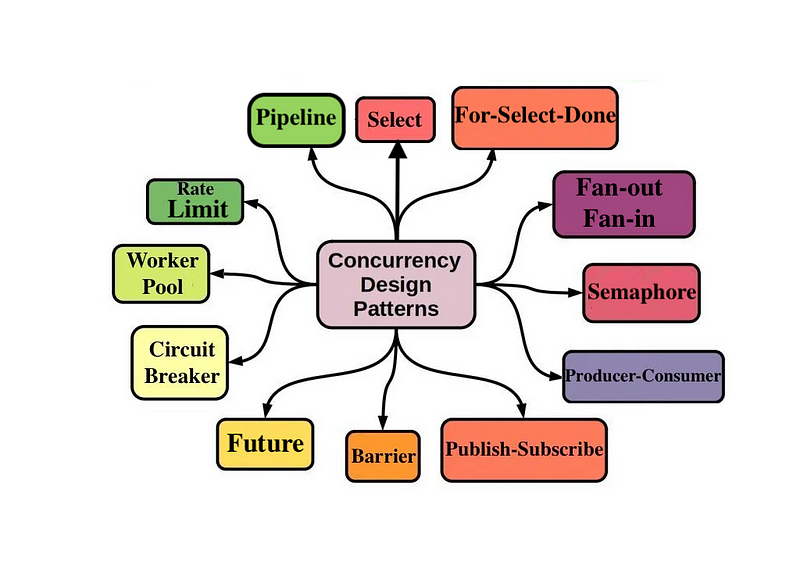
These patterns are essential for efficiently and safely executing multiple tasks simultaneously. Go's robust support for concurrency, via Goroutines and Channels, makes it a favored choice for concurrent programming.
Select Pattern
The Select pattern in Go is a control flow mechanism that enables a goroutine to wait for multiple channel operations and execute different code depending on which channel becomes ready first. This pattern is commonly employed for implementing timeouts, multiplexing input from various channels into a single channel, or performing non-blocking operations on channels. It serves as a crucial tool for managing concurrent tasks in Go and is fundamental to many concurrency designs.
For a deeper understanding, you can watch this video:
For-Select-Done Pattern
The For-Select-Done pattern is a prevalent idiom in Go that merges the for loop with the select statement, allowing for continuous monitoring of events until a "done" signal is received. This approach is particularly useful for managing asynchronous tasks in a non-blocking way.
Future Pattern
The Future pattern in Golang involves initiating a goroutine to compute a value that will be required later, immediately returning a channel where the computed value will be sent once it's ready. This method allows computations to occur concurrently with other tasks, avoiding the need to block the current goroutine.
For additional insights, check out this video:
Barrier Pattern
The Barrier pattern acts as a synchronization mechanism that allows goroutines to wait for one another. It is often applied in parallel algorithms that divide a problem into independent sub-problems that can be solved concurrently. Once a goroutine completes its assigned task, it reaches the barrier and waits until all goroutines have reached the same point, at which point they can all proceed together.
Pipeline Pattern
The Pipeline pattern in Go is a powerful technique for constructing a series of processing elements in which the output of one element serves as the input to the next. This is frequently used in data processing tasks to segment an operation on a data sequence into individual stages that can run concurrently. Each stage consists of one or more goroutines that communicate via channels, enhancing efficiency and performance in applications involving a series of concurrent operations.
Worker Pool Pattern
The Worker Pool pattern involves a group of goroutines (workers) that listen to a single task channel. This structure is advantageous for regulating the number of concurrently executing goroutines and maintaining control over the concurrency level, especially in scenarios with numerous tasks needing processing.
Semaphore Pattern
The Semaphore pattern is a synchronization primitive in concurrent programming that manages access to shared resources. Although Go lacks a built-in semaphore type, it offers an implementation using channels, which are integral to Go's concurrency framework.
Producer-Consumer Pattern
The Producer-Consumer pattern is a classic concurrency structure where one or more goroutines (producers) generate data, while one or more goroutines (consumers) utilize that data. In Go, this is typically realized through goroutines for both producers and consumers, with channels facilitating data transfer between them, enabling a decoupling of data production from consumption.
Fan-Out Fan-In Pattern
The fan-out pattern initiates multiple goroutines to concurrently handle input from a single channel, enhancing throughput and allowing for distributed computation. Conversely, the fan-in pattern merges multiple results into a single channel, which is useful for aggregating outputs from several goroutines performing similar tasks.
Rate Limit Pattern
The Rate Limit pattern is a technique for controlling the frequency of actions, such as requests to a server or method calls. In Go, this can be implemented using a ticker and a buffered channel, where each tick signifies a “permit” to act.
Circuit Breaker Pattern
The Circuit Breaker pattern is a design approach used in modern software to identify failures and encapsulate logic that prevents a failure from recurring. This is beneficial during maintenance, temporary external failures, or unexpected difficulties, allowing the application to avoid operations likely to fail.
Publish-Subscribe Pattern
The Publish-Subscribe pattern is a messaging architecture where message senders (publishers) do not send messages directly to specific receivers (subscribers). Instead, published messages are categorized into structures, and subscribers express interest in these structures, receiving only relevant messages.
Scenarios for Applying Concurrency Patterns
Different concurrency patterns are suited for various scenarios:
- Coordinate multiple goroutines using the Worker Pool and Semaphore patterns.
- Create processing stages with the Pipeline pattern.
- Handle multiple channel operations with the Select and For-Select-Done patterns.
- Boost throughput with the Fan-Out Fan-In patterns.
- Manage data production and consumption using the Producer-Consumer pattern.
- Control action rates through the Rate Limit pattern.
- Prevent recurring failures with the Circuit Breaker pattern.
- Compute future values with the Future pattern.
- Synchronize goroutines using the Barrier pattern.
- Broadcast messages to interested parties with the Publish-Subscribe pattern.
Conclusion
The concurrency patterns in Go, including Select, For-Select-Done, Future, Barrier, Pipeline, Worker Pool, Fan-Out Fan-In, Producer-Consumer, Semaphore, Rate Limit, Circuit Breaker, and Publish-Subscribe, provide essential tools for effectively managing concurrent executions and data flows in Go applications.
For more insights into design patterns in Golang, explore the following links:
- [Creational Design Patterns in Golang](#)
- [Structural Design Patterns in Golang](#)
- [Behavioral Design Patterns in Golang](#)
- [Concurrency Design Patterns in Golang](#)
- [Microservices Design Patterns in Golang](#)