Getting Started with Pub/Sub: A Beginner's Guide Using Python
Written on
Chapter 1: Introduction to Pub/Sub
The world of message queues has been integral to software development for many years. This approach to transferring messages between various system components has been a fundamental aspect of many programmers' work. If you're new to message queues or eager to experiment with a different protocol, this article is an ideal place to begin.
Utilizing message queues can significantly enhance application scalability. This method allows you to minimize overhead while making your code more adaptable and robust. In this guide, we will explore a widely-used messaging model known as publish-subscribe, or pub/sub. We will set up message queues via a Redis server and interact with them using a Python client.
Creating a pub/sub system with these two elements is straightforward, enabling you to get started in just a few minutes. Let's dive into the setup process.
Section 1.1: Setting Up Redis
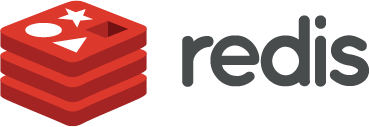
Redis serves as a high-speed, in-memory database and functions as a versatile key/value store suitable for various applications, including caching and temporary data storage. For our project, we simply need to run a basic Redis server accessible on a specific port without modifying configuration files or performing database migrations.
Instead of installing Redis directly, we can deploy it using Docker with the following command:
docker run --name my-redis -p 6379:6379 -d redis
Upon executing this, you should have Redis running as a container with the default port 6379 available.
For additional information on different Docker image variations, visit the official Redis Docker Hub:
Section 1.2: Understanding Topics
In the context of pub/sub, a topic can be likened to a room. Various clients can subscribe to a topic, allowing them to receive all messages sent to that room. A client can be part of multiple rooms and can join or leave as desired. The server simply manages the publication of messages to each topic, ensuring that all clients receive the broadcast messages without needing to service each subscriber individually.
To learn more about pub/sub architecture and topics, check out:
Chapter 2: Implementing a Python Publisher
To connect to the Redis server from Python and begin publishing data to a topic, create a new file called publisher.py and include the following code:
# publisher.py
from time import sleep, time
from redis import Redis
redis = Redis(host='127.0.0.1', port=6379)
while True:
redis.publish('topic1', f'unix time is: {time()}')
sleep(1)
Let’s dissect this step-by-step:
- Import the Redis module and time functions.
- Establish a connection to the local Redis server.
- Initiate an infinite loop.
- Publish the current Unix time to topic1.
- Pause for one second before repeating the loop.
Running this script will not yield any visible output, as it directly publishes to the topic. The output will appear on the subscriber's end. This basic example could be adapted for various applications, such as usage metrics or live performance data.
Section 2.1: Setting Up a Python Subscriber
With data flowing into our topic, we can now subscribe and start receiving messages. Create a new file named subscriber.py and add the following code:
# subscriber.py
from redis import Redis
redis = Redis(host='127.0.0.1', port=6379)
pubsub = redis.pubsub()
pubsub.subscribe('topic1')
for msg in pubsub.listen():
print(msg)
Breaking down this code:
- Import the Redis module and connect to the local server.
- Create a new pubsub client object.
- Subscribe to topic1.
- Listen for incoming messages in an infinite loop and print them out.
This listen() function blocks and continually loops, waiting for messages. If you prefer to receive a single message, you can use the get_message method instead.
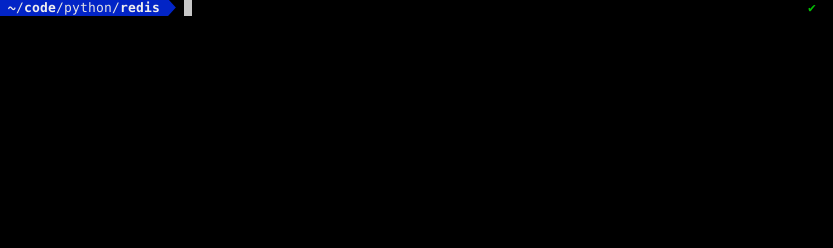
Section 2.2: Enhancing Functionality with Threading
To allow other actions while subscribing or publishing to a topic, you can implement threading as follows:
from threading import Thread
from time import sleep, time
from redis import Redis
redis = Redis(host='127.0.0.1', port=6379)
def server():
while True:
redis.publish('topic1', f'unix time is: {time()}')
sleep(1)
def client():
pubsub = redis.pubsub()
pubsub.subscribe('topic1')
while True:
msg = pubsub.get_message()
if msg:
print(msg)sleep(0.001)
server_thread = Thread(target=server)
client_thread = Thread(target=client)
server_thread.start()
client_thread.start()
In this script, both the publisher and subscriber are defined within their own functions and executed in separate threads. This allows you to run the publisher and subscriber concurrently, facilitating seamless message handling.
Chapter 3: Conclusion
As demonstrated, establishing a basic pub/sub system with these components is remarkably simple. You only require a small amount of code and a functioning Redis server. The remainder is up to your creativity and application requirements.
For more comprehensive information on using the Redis module for Python, refer to the official documentation:
Thank you for taking the time to read this guide! If you haven't yet, please consider subscribing and following for more insightful content.
Looking for additional resources? Explore these articles:
- 5 Linux Time Utilities You Need To Know
- 6 Special Linux Device Files And Their Uses
- How and When to Use Context Managers in Python
- 10 More Python Methods for Manipulating Strings
Chapter 4: Video Tutorials
To further enhance your understanding, check out these related YouTube videos:
First, watch "Set up & use PubSub with Python" for a comprehensive overview on setting up Pub/Sub in Python.
Next, delve into "RabbitMQ - Tutorial 10a - Pub/Sub Python Implementation" to see a practical implementation of the pub/sub pattern using RabbitMQ.