Exploring Race Conditions in Java: A Closer Look
Written on
Chapter 1: Understanding Race Conditions
Race conditions are a significant challenge in concurrent programming, often leading to unexpected behavior in applications. The term "Nice Race Condition" might seem contradictory, but it reflects the intriguing nature of these issues, which can appear innocuous on the surface while hiding complex problems underneath. This phenomenon serves as an important case study demonstrating how seemingly simple programs can encounter failures in multi-threaded environments.
In this discussion, we will analyze a specific race condition, delving into its causes and exploring methods to mitigate its impact on our applications.
Let us begin...
Consider the following Java code snippet:
public class NiceRC {
public static void main(String[] args) {
final Map<Object, Object> unsafeMap = new HashMap<>();
final List<Thread> threads = new ArrayList<>();
for (int i = 0; i < 5; ++i) {
threads.add(new Thread(() -> {
for (int j = 0; j < 100000; ++j) {
unsafeMap.put(new Object(), new Object());}
}));
}
threads.forEach(Thread::start);
threads.forEach(thread -> {
try {
thread.join();} catch (InterruptedException e) {
e.printStackTrace();}
});
System.out.println("Size of unsafeMap: " + unsafeMap.size());
}
}
It is essential to thoroughly review the code above before moving forward. In this example, we initiate five threads, each of which adds approximately 100,000 entries to a shared map called unsafeMap. If you execute this code multiple times, you'll eventually encounter a scenario where the code fails to complete, seemingly entering an infinite loop. But why does this happen? There are no explicit loops in the code.
The culprit here is a race condition.
To better understand this issue, we need to revisit Java's HashMap, as comprehending its internals is crucial to grasping the underlying problems.
HashMap’s Internal Structure
HashMap is a hash table-based implementation of the Map interface. It utilizes a hash function to assign keys to specific buckets, where the corresponding values can be accessed by traversing a linked list.
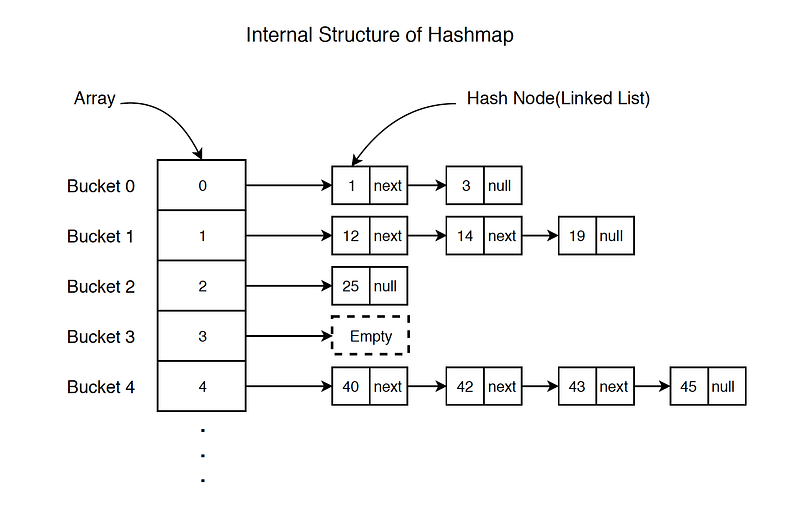
The Entry class represents the nodes within the linked list of a HashMap:
static class Entry<K,V> implements Map.Entry<K,V> {
final K key;
V value;
Entry<K,V> next;
int hash;
}
When the count of key-value pairs in a HashMap surpasses a certain threshold, it must resize its internal array and rehash all existing elements. This operation is known as rehashing or resizing.
Here is an excerpt of the code responsible for this resizing operation:
// Transfer method in java.util.HashMap - called to resize the hashmap
for (int j = 0; j < src.length; j++) {
Entry e = src[j];
if (e != null) {
src[j] = null;
do {
Entry next = e.next;
int i = indexFor(e.hash, newCapacity);
e.next = newTable[i];
newTable[i] = e;
e = next;
} while (e != null);
}
}
Understanding Rehashing
To illustrate how this rehashing works, consider a scenario with two buckets and three elements.

During a resizing operation, the number of buckets is increased to four, and all three map elements must be rehashed. Keys A and B will be hashed to Bucket 3, while key C will go to Bucket 1.
After one pass through the loop, the map's state will change as follows:
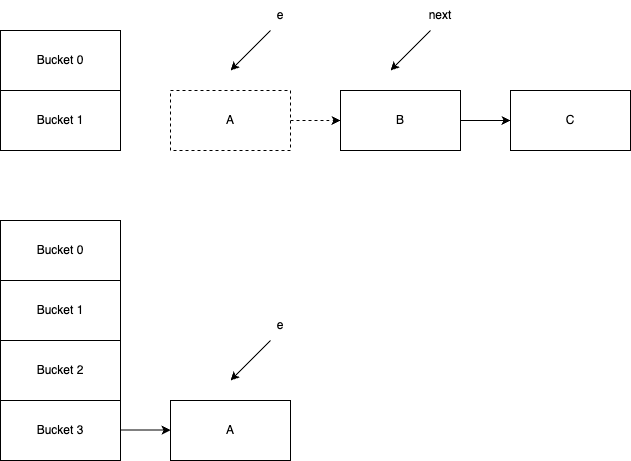
Following additional iterations, the state of the map will evolve further.
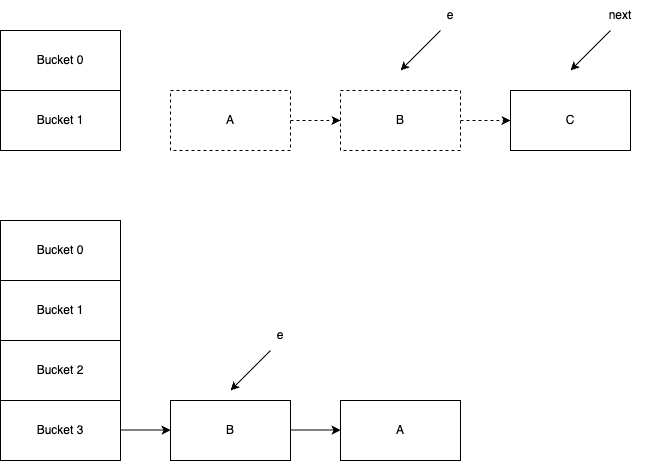
Once the rehashing process is complete, the final state of the map will appear as follows:
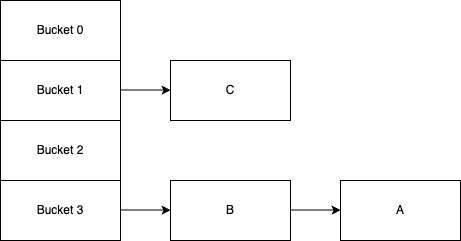
Diving into the Race Condition
With a solid understanding of HashMap's internals, we can now identify the race condition affecting our NiceRC class. Let's revisit the earlier map example with two buckets and three elements.

Imagine that two threads, referred to as Thread 1 and Thread 2, attempt to perform the resizing operation simultaneously. We will examine how the interleaving of these operations could result in an infinite loop.
Consider Thread 1 entering the resizing loop first. It initializes its local variables and then, for some unknown reason, gets preempted by the CPU.
// Transfer method in java.util.HashMap - called to resize the hashmap
for (int j = 0; j < src.length; j++) {
Entry e = src[j];
if (e != null) {
src[j] = null;
do {
Entry next = e.next;
// THREAD 1 STOPS HERE
int i = indexFor(e.hash, newCapacity);
e.next = newTable[i];
newTable[i] = e;
e = next;
} while (e != null);
}
}
At this moment, Thread 1 holds its local variables, while Thread 2 continues and completes the resizing process. The state of the map now changes.
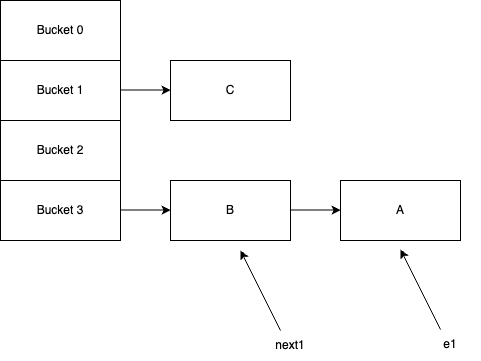
Thread 1 resumes execution, still pointing to its initial variables, which now reference objects in the altered state of the map. The next pointers have been modified, resulting in a corruption of the map's structure.
As Thread 1 attempts to add node A to the new bucket, it inadvertently creates a loop due to the outdated references.
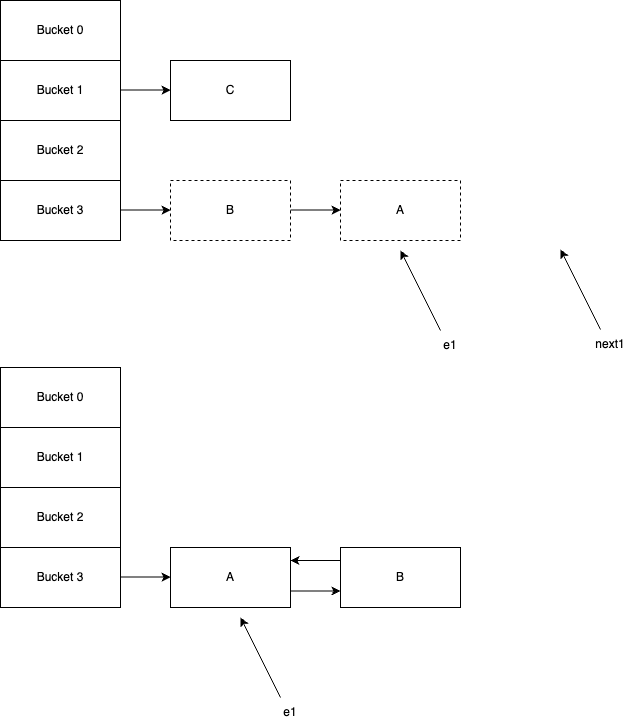
Consequently, any further operations targeting this corrupt bucket will be trapped in an infinite loop. This is precisely what occurred in our NiceRC class.
Working with non-thread-safe classes in a concurrent context introduces significant risks. Even if your system can manage race conditions, unexpected interleaving of operations can still lead to failures. Thus, it is advisable to utilize thread-safe implementations for data structures in multi-threaded environments.
If you found this analysis insightful, feel free to show your appreciation!
Additional Resources
Consider exploring these related articles on concurrency:
- Object Sharing in Multi-Threaded Environments
- Visibility Across Threads
- Thread Safety from an Object-Oriented Perspective
- Java’s Synchronized Collections
- Producer-Consumer Pattern Using Java’s Blocking Queue
Finally, for further reading, check out the original blog post that inspired this article.
Chapter 2: Additional Insights on Race Conditions
The first video, titled "What is a Race Condition? (and how to exploit it)," provides a comprehensive overview of race conditions, including practical demonstrations.
The second video, "What are Race Conditions? Race Conditions Explained with Real Examples & Go Code," offers real-world examples to clarify the concept of race conditions further.